Axioned’s React Best Practices
Facebook’s React is a declarative, efficient, and flexible JavaScript library for building user interfaces (UI).
When I first began writing code in React I remember seeing many different approaches varying greatly from tutorial to tutorial. So my team at Axioned and I came up with a guide/list of best practices (BPs) that would help us all get up to speed, faster.
The following is our guide. We hope this helps you speed-up your adoption and usage of React; whether you’re just starting out/a beginner or an experienced developer.
1. Maintain an Organized Folder Structure:
As with any programming/coding project, this is very important! If your project files are not organized logically, you and your collaborators can get confused and waste precious time looking for files or editing the wrong files.
At Axioned we came up with the following folder structure to make things easier and less frustrating for everyone. Click play to watch.
React Folder Structure: So important!
2. Components — Chunks & Trees. Hey, you’re a Javascript Lumberjack!
Components are the heart of a React. A React component is basically any part of a UI.
Create logical UI chunks (groupings): Always divide your components into chunks (aka logical groupings). Consider any application interface and start dividing the UI into smaller logical chunks. Each of these UI chunks (groups) are potential components.
Tree, Branches and Sub-Branches: The complete/full view of the UI (the tree or trunk) is divided into logical chunks (aka branches). The tree becomes the starting component (a layout component) and then each chunk in the UI (aka branch) will become a sub-component that can be further divided into sub-components (aka sub-branches). This keeps the UI organized and also allows data and state changes to logically flow from the tree to the branches, and then the sub-branches.
3. Components — Functional and Class-Based:
There are two types of components: functional and class-based.
When should I go with a functional component? Go with a functional component if your component doesn’t do much more than rendering props. Think of functional components as pure functions: they will always render the same way and behave the same way, given the same props. Also, they don’t care about life-cycle methods; they are stateless components.

When should I go with a class-based component? If your component needs internal state and component lifecycle methods then go for a class based component. Check this out to help you understand more about “internal state” and “lifecycle methods”.
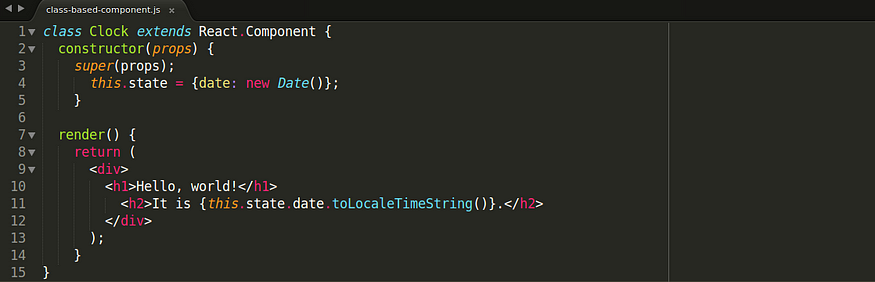
4. Props! Props to you! Props to me! But no, we’re talking React Props!
“Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen.” Click for source.
Bring in the Camels! Nah, you can leave those camels in the dessert. We’re talking camelCase here. Always use camelCase for prop names. Props can also be viewed as attributes, and the convention set by React is to use camelCase for JSX attributes.

Believe me, it’s true, I swear! Omit the value of the prop when it is explicitly true. Even if we don’t assign a true value to a prop it’s considered a true value, so there’s no need to assign ‘true’ as a value to the prop.

Avoid using an array index as key prop; use a unique ID instead: We’ve seen too many developers use the index of an item as its key when they render a list, which is SO not good! This is what they incorrectly write…

A key is the only thing React uses to identify DOM elements. So what happens if you push an item to the list or remove something from the middle? If the key is the same as before, React assumes that the DOM element represents the same set of components (as before). But this is not the case!
This is why you should use a unique ID. Each item should have a permanent and unique property (ID). And ideally it (the ID) should be assigned when the item is created. So it’s written out something like this…
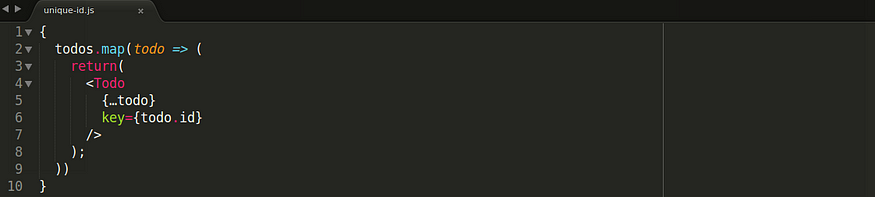
Always define explicit defaultProps for all non-required props. Providing defaultProps means the reader of your code doesn’t have to assume things, because they’ll know the default value for the prop just by looking at defaultProps while rendering components.

As you can see, there are small but very important things that go into making your React code relatively error free during the review process. Spending the time upfront to learn these best practices, and use them, means you spend less time re-writing code to fix your mistakes, giving you more time to do the things you love. And if doing the things you love means writing code, you’ll get more time to write code for a different project!
More of Axioned’s React best practices can be found here on Github: https://github.com/axioned. Please feel free to share and contribute to this list. And if you have any issues in accessing, hit us up in the comments here.
Interested in working with Axioned as a client or as an employee? Then hit us up! Go here www.axioned.com for further details, including contact details.